Convert Int To String Dev C++
There are three ways of converting an integer into a string:
- By using stringstream class
- By using to_string() method
- By using boost.lexical cast
C library function - atoi - The C library function int atoi(const char.str) converts the string argument str to an integer (type int).
The stringstream class. As mentioned earlier, C stringstream class associates the object (string object) with the stream of the input provided.It can be used to convert the type of the input stream from integer to string and vice-versa. Aug 17, 2017 For gameplay programmers writing C code.
Conversion of an integer into a string by using stringstream class.
The stringstream class is a stream class defined in the
The following are the operators used to insert or extract the data:
- Operator >>: It extracts the data from the stream.
- Operator <<: It inserts the data into the stream.
Let's understand the concept of operators through an example.
- In the below statement, the << insertion operator inserts the 100 into the stream.
stream1 << 100; - In the below statement, the >> extraction operator extracts the data out of the stream and stores it in 'i' variable.
stream1 >> i;
Let's understand through an example.
Output
In the above example, we created the k variable, and want to convert the value of k into a string value. We have used the stringstream class, which is used to convert the k integer value into a string value. We can also achieve in vice versa, i.e., conversion of string into an integer value is also possible through the use of stringstream class only.
Let's understand the concept of conversion of string into number through an example.
Output
Conversion of an integer into a string by using to_string() method.
The to_string() method accepts a single integer and converts the integer value or other data type value into a string.
Let's understand through an example:
Output
Conversion of an integer into a string by using a boost.lexical cast.
The boost.lexical cast provides a cast operator, i.e., boost.lexical_cast which converts the string value into an integer value or other data type value vice versa.
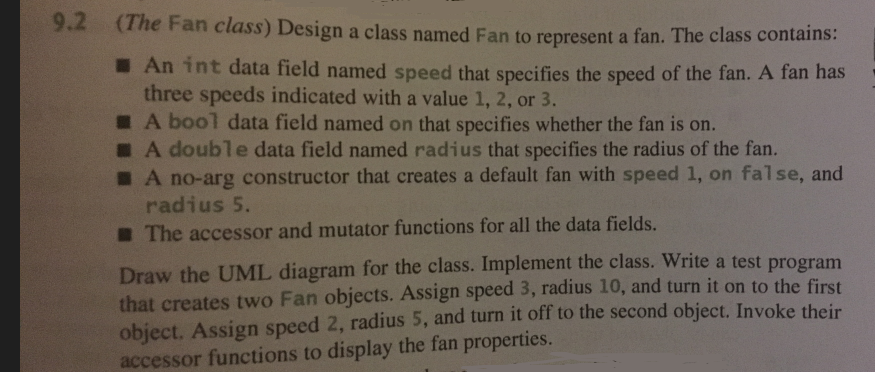
Let's understand the conversion of integer into string through an example.
Output
In the above example, we have converted the value of 'i' variable into a string value by using lexical_cast() function.
Let's understand the conversion of string into integer through an example.
Output

In the above example, we have converted the string value into an integer value by using lexical_cast() function.
We use g++ compiler to turn provided C code into assembly language. To see the assembly code generated by the C compiler, we can use the “-S” option on the command line:
Syntax:
This will cause gcc to run the compiler, generating an assembly file. Suppose we write a C code and store it in a file name “geeks.c” .
#include <stdio.h> // global string int main() // Declaring variables printf ( '%s %d' , s, a+b); |
Running the command:
Convert Int To String In C
This will cause gcc to run the compiler, generating an assembly file geeks.s, and go no further. (Normally it would then invoke the assembler to generate an object- code file.)
The assembly-code file contains various declarations including the set of lines:
.section __TEXT, __text, regular, pure_instructions .globl _main _main: ## @main ## BB#0: Ltmp0: Ltmp1: movq %rsp, %rbp .cfi_def_cfa_register %rbp leaq L_.str(%rip), %rdi movl $2000, -4(%rbp) ## imm = 0x7D0 movl -4(%rbp), %eax movl %eax, %edx callq _printf movl %eax, -12(%rbp) ## 4-byte Spill addq $16, %rsp retq .globl _s ## @s .asciz 'GeeksforGeeks' .section __TEXT, __cstring, cstring_literals .asciz '%s %d' |
Guitar vst plugins free download standalone. Each indented line in the above code corresponds to a single machine instruction. For example, the pushq instruction indicates that the contents of register %rbp should be pushed onto the program stack. All information about local variable names or data types has been stripped away. We still see a reference to the global
variable s[]= “GeeksforGeeks”, since the compiler has not yet determined where in memory this variable will be stored.
C++ Convert String Into Int
Please write comments if you find anything incorrect, or you want to share more information about the topic discussed above.